Fall 2024 - P1
Big Idea 3 | .1 | .2 | .3 | .4 | .5 | .6 | .7 | .8 | .10 |
3.4 String Operations
3.4 Team Teach String Operations
- Measuring String Length
- Measuring String Length
- String Case Convertion
- String Slicing
- Finding Substrings
- Replacing Substrings
- Splitting Strings
- Joining Strings
- Sample Hack - String Analyzation
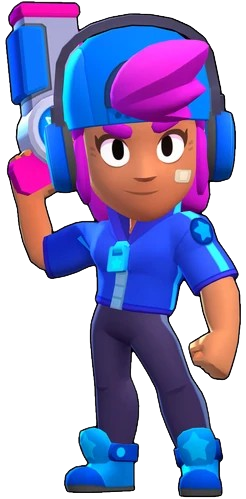
____________________________________________________________________________________________________________________________________________
Measuring String Length
</body>
</html>
Measuring String Length
- The len() function in Python allows us to find the length of a string
- Use .length in JavaScript to do this as well
%%js
console.log("Hello".length);
<IPython.core.display.Javascript object>
String Case Convertion
- Uppercase: Convert to uppercase using .upper() in Python, .toUpperCase() in JS
- Lowercase: Convert to lowercase using .lower() in Python, .toLowerCase() in JS
%%js
console.log("hello".toUpperCase());
console.log("HELLO".toLowerCase());
<IPython.core.display.Javascript object>
String Slicing
- Through string slicing, we can access a part of the string using indexes
- Each character in a string gets assigned a index, starting from 0
- Syntax in Python is [startindex:endindex]
- Syntax in JS is .slice(startindex, endindex)
%%js
console.log("Hello Brawler".slice(0, 5));
<IPython.core.display.Javascript object>
Finding Substrings
- Searches for a substring and returns its position within a overlaying string
- .find() in Python
- .indexOf() in JS
%%js
console.log("Hello Brawler".indexOf("Brawler"));
<IPython.core.display.Javascript object>
Replacing Substrings
- Can replace different parts of a string with something else
- .replace() in Python and JS
%%js
console.log("Hello Brawler".replace("Brawler", "Mihir"));
<IPython.core.display.Javascript object>
Splitting Strings
- Splits a string into a list of substrings based on a delimiter (most commonly a space or comma)
- .split() in Python and JS
%%js
console.log("Darryl,Mico,Fang".split(","));
<IPython.core.display.Javascript object>
Joining Strings
- Basically opposite of splitting strings
- .join() in Python and JS
%%js
console.log(['Darryl', 'Mico', 'Fang'].join(","));
<IPython.core.display.Javascript object>
Sample Hack - String Analyzation
- Determine metrics about strings (length, chars, palidrome(?), etc.)
%%js
const string1 = "Brawl Stars is a fun game!";
const string2 = "Star Brawl war Brats";
console.log("String 1: " + string1);
const length1 = string1.length;
console.log("Length: " + length1);
function countVowels(inputString) {
const vowels = 'aeiouAEIOU';
let count = 0;
for (const char of inputString) {
if (vowels.includes(char)) {
count++;
}
}
return count;
}
console.log("Vowel Count: " + countVowels(string1));
function averageWordLength(inputString) {
const words = inputString.split(/\s+/);
if (words.length === 0) {
return 0;
}
const totalLength = words.reduce((sum, word) => sum + word.length, 0);
return totalLength / words.length;
}
console.log("Average Word Length: " + averageWordLength(string1));
function isPalindrome(inputString) {
const sanitizedString = inputString.replace(/\s+/g, '').toLowerCase();
return sanitizedString === sanitizedString.split('').reverse().join('');
}
console.log("Palindrome or Not? " + isPalindrome(string1));
console.log("String 2: " + string2);
const length2 = string2.length;
console.log("Length: " + length2);
console.log("Vowel Count: " + countVowels(string2));
console.log("Average Word Length: " + averageWordLength(string2));
console.log("Palindrome or Not? " + isPalindrome(string2));
<IPython.core.display.Javascript object>