Fall 2024 - P1
Big Idea 3 | .1 | .2 | .3 | .4 | .5 | .6 | .7 | .8 | .10 |
3.1 Variables and Strings
Team Teach String Operations
- Homework Hack:
- In this hack, you will be given a set of personal information, and your job is to format and display it properly, along with generating a unique ID associated to that person using string slicing and concatenation.
- Data Set:
- Recreate as JavaScript, with different parameters, a different/unique method of creating an ID, and a different way to display/store the data
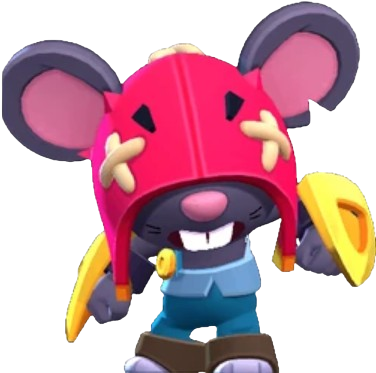
____________________________________________________________________________________________________________________________________________
Homework Hack:
In this hack, you will be given a set of personal information, and your job is to format and display it properly, along with generating a unique ID associated to that person using string slicing and concatenation.
Data Set:
full_name = "John Doe"
age = 28
email = "john.doe@gmail.com"
hobby = "Food Tasting"
dietary_preferences = "Vegan"
initials = full_name[0] + full_name[full_name.find(" ") + 1]
unique_id = initials + str(age)
formatted_info = "Personal Info:\n" \
"- Full Name: " + full_name + "\n" \
"- Age: " + str(age) + "\n" \
"- Email: " + email + "\n" \
"- Hobby: " + hobby + "\n" \
"- Dietary Preferences: " + dietary_preferences + "\n" \
"- Unique ID: " + unique_id
print(formatted_info)
Personal Info:
- Full Name: John Doe
- Age: 28
- Email: john.doe@gmail.com
- Hobby: Food Tasting
- Dietary Preferences: Vegan
- Unique ID: JD28
Your Goal:
Recreate as JavaScript, with different parameters, a different/unique method of creating an ID, and a different way to display/store the data
</body>