Fall 2024 - P5
Big Idea 3 | .1 | .2 | .3 | .4 | .5 | .6 | .7 | .8 | .10 |
3.7 Nested Conditionals
Student led teaching on Conditionals.
Nested Conditionals
Introduction
Nested conditionals are essentially conditionals inside conditionals. They can be used when you’re creating conditionals that can have multiple possible situations.
Flowchart Example
This is a flowchart example using draw.io explainig how nested conditionals generally work
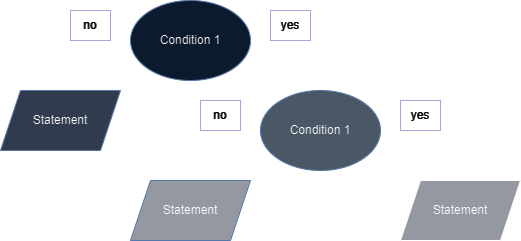
Python example
In this example, the nested conditional statement checks the budget and depending on how much money there is, it will determine what kind of iphone can be purchased.
%%python
# Budget
budget = 900
# Phone prices
iphone_16_price = 1200
iphone_14_price = 1000
iphone_12_price = 800
# Determine which iPhone you can buy
if budget >= iphone_16_price:
print("You can buy an iPhone 16!")
elif budget >= iphone_14_price:
print("You can buy an iPhone 14!")
elif budget >= iphone_12_price:
print("You can buy an iPhone 12!")
else:
print("You don't have enough money to buy an iPhone.")
You can buy an iPhone 12!
In the example, my budget is $900. The statement means that if the budget price is t least $1200 (iphone 16 price), then it will print “You can buy an iPhone 16!” Since that is not true for my budget, it will keep going through the conditional statements until it finds one that applies to my situation. In this case, it is the second to last condition, which is the price of the iPhone 12. Therefore, it should print out “You can buy an iPhone 12!”
Popcorn Hack
Change the set budget in the iphone example to see what other messages will be printed out.
Javascript example
Here is the same example but now in Javascript
%%js
// Budget
let budget = 900;
// Phone prices
let iphone_16_price = 1200;
let iphone_14_price = 1000;
let iphone_12_price = 800;
// Determine which iPhone you can buy
if (budget >= iphone_16_price) {
console.log("You can buy an iPhone 16!");
} else if (budget >= iphone_14_price) {
console.log("You can buy an iPhone 14!");
} else if (budget >= iphone_12_price) {
console.log("You can buy an iPhone 12!");
} else {
console.log("You don't have enough money to buy an iPhone.");
}
<IPython.core.display.Javascript object>
Example 2
Here is another example but instead of numerical values as the variable conditions, it is true or false. This means that the script checks for whether or not the variable exists in this situation.
Python Example
%%python
# Ingredients
has_flour = True
has_sugar = True
has_eggs = True
has_milk = False
has_baking_powder = True
has_vanilla_extract = False
# Baking logic based on available ingredients
if has_flour and has_sugar:
print("You have the basic ingredients.")
if has_eggs:
print("You can make a basic cake batter.")
if has_milk:
print("Great! The cake will be moist.")
else:
print("You don't have milk, the cake might be a bit dry.")
if has_baking_powder:
print("Your cake will rise perfectly.")
else:
print("Without baking powder, the cake might not rise.")
if has_vanilla_extract:
print("The cake will have a nice vanilla flavor.")
else:
print("No vanilla extract, the flavor will be more basic.")
else:
print("You can't bake a cake without eggs.")
else:
print("You don't have enough ingredients to bake a cake.")
You have the basic ingredients.
You can make a basic cake batter.
You don't have milk, the cake might be a bit dry.
Your cake will rise perfectly.
No vanilla extract, the flavor will be more basic.
Popcorn Hack
This code has many different “ingredients” in it. Change up which ingredients are true or false in order to get new printed messages.
Javascript Example
This is the same code but in JavaScript
%%js
// Ingredients
let has_flour = true;
let has_sugar = true;
let has_eggs = true;
let has_milk = false;
let has_baking_powder = true;
let has_vanilla_extract = false;
// Baking logic based on available ingredients
if (has_flour && has_sugar) {
console.log("You have the basic ingredients.");
if (has_eggs) {
console.log("You can make a basic cake batter.");
if (has_milk) {
console.log("Great! The cake will be moist.");
} else {
console.log("You don't have milk, the cake might be a bit dry.");
}
if
<IPython.core.display.Javascript object>
Hacks
Hack 1
Now it’s time to practice Nested Conditionals!
- test out the examples above and change up the the starting values to play with how the conditions within eachother work
- demonstrate knowledge of nested conditionals by creating your own nested conditional statements.